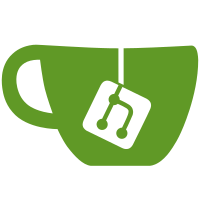
Signed-off-by: Izuru Yakumo <yakumo.izuru@chaotic.ninja> git-svn-id: file:///srv/svn/repo/yukari/trunk@152 f3bd38d9-da89-464d-a02a-eb04e43141b5
37 lines
1.1 KiB
Go
37 lines
1.1 KiB
Go
package config
|
|
|
|
import (
|
|
"gopkg.in/ini.v1"
|
|
)
|
|
|
|
var Config struct {
|
|
Debug bool
|
|
ListenAddress string
|
|
Key string
|
|
IPV6 bool
|
|
RequestTimeout uint
|
|
FollowRedirect bool
|
|
MaxConnsPerHost uint
|
|
UrlParameter string
|
|
HashParameter string
|
|
ProxyEnv bool
|
|
}
|
|
|
|
func ReadConfig(file string) error {
|
|
cfg, err := ini.Load(file)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
Config.Debug, _ = cfg.Section("yukari").Key("debug").Bool()
|
|
Config.ListenAddress = cfg.Section("yukari").Key("listen").String()
|
|
Config.Key = cfg.Section("yukari").Key("key").String()
|
|
Config.IPV6, _ = cfg.Section("yukari").Key("ipv6").Bool()
|
|
Config.RequestTimeout, _ = cfg.Section("yukari").Key("timeout").Uint()
|
|
Config.FollowRedirect, _ = cfg.Section("yukari").Key("followredirect").Bool()
|
|
Config.MaxConnsPerHost, _ = cfg.Section("yukari").Key("max_conns_per_host").Uint()
|
|
Config.UrlParameter = cfg.Section("yukari").Key("urlparam").String()
|
|
Config.HashParameter = cfg.Section("yukari").Key("hashparam").String()
|
|
Config.ProxyEnv, _ = cfg.Section("yukari").Key("proxyenv").Bool()
|
|
return nil
|
|
}
|