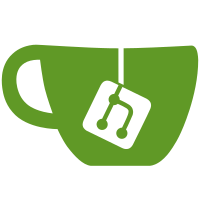
Signed-off-by: Izuru Yakumo <yakumo.izuru@chaotic.ninja> git-svn-id: https://svn.yakumo.dev/yakumo.izuru/kosuzu/trunk@71 eb64cd80-c68d-6f47-b6a3-0ada418499da
45 lines
913 B
Go
45 lines
913 B
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
)
|
|
|
|
var (
|
|
authors string
|
|
id string
|
|
title string
|
|
)
|
|
|
|
func main() {
|
|
fmt.Print("Name of the repository: ")
|
|
fmt.Scanln(&title)
|
|
fmt.Print("Base32 unique identifier (must be 6 characters long): ")
|
|
fmt.Scanln(&id)
|
|
fmt.Print("Authors (addresses in the <protocol:name@example.com> format: ")
|
|
fmt.Scanln(&authors)
|
|
|
|
f, err := os.Create("./txt.conf")
|
|
if err != nil {
|
|
fmt.Println("Error creating txt.conf")
|
|
os.Exit(1)
|
|
}
|
|
defer f.Close()
|
|
|
|
pwd, err := os.Getwd()
|
|
if err != nil {
|
|
fmt.Println("Unable to get working directory")
|
|
os.Exit(1)
|
|
}
|
|
|
|
f.WriteString("Id: " + fmt.Sprint(id) + "\n")
|
|
f.WriteString("Title: " + fmt.Sprint(title) + "\n")
|
|
f.WriteString("Authors: " + fmt.Sprint(authors) + "\n")
|
|
|
|
fmt.Println("All done!")
|
|
fmt.Println("Configuration file written to txt.conf")
|
|
fmt.Println("Read the documentation in Logarion's repository")
|
|
fmt.Printf("%v\n", pwd)
|
|
}
|
|
|