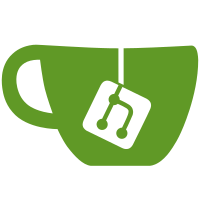
Signed-off-by: Izuru Yakumo <yakumo.izuru@chaotic.ninja> git-svn-id: file:///srv/svn/repo/aya/trunk@88 cec141ff-132a-4243-88a5-ce187bd62f94
58 lines
1.2 KiB
Go
58 lines
1.2 KiB
Go
// Build everything and store it on PUBDIR
|
|
// If boolean watch is true, it keeps on going
|
|
// every time you modify something.
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"path/filepath"
|
|
"strings"
|
|
"time"
|
|
)
|
|
|
|
func buildAll(watch bool) {
|
|
lastModified := time.Unix(0, 0)
|
|
modified := false
|
|
|
|
vars := globals()
|
|
for {
|
|
os.Mkdir(PUBDIR, 0755)
|
|
filepath.Walk(".", func(path string, info os.FileInfo, err error) error {
|
|
// ignore hidden files and directories
|
|
if filepath.Base(path)[0] == '.' || strings.HasPrefix(path, ".") {
|
|
return nil
|
|
}
|
|
// inform user about fs walk errors, but continue iteration
|
|
if err != nil {
|
|
fmt.Println("error:", err)
|
|
return nil
|
|
}
|
|
|
|
if info.IsDir() {
|
|
os.Mkdir(filepath.Join(PUBDIR, path), 0755)
|
|
return nil
|
|
} else if info.ModTime().After(lastModified) {
|
|
if !modified {
|
|
// First file in this build cycle is about to be modified
|
|
run(vars, "prehook")
|
|
modified = true
|
|
}
|
|
fmt.Println("GEN", path)
|
|
return build(path, nil, vars)
|
|
}
|
|
return nil
|
|
})
|
|
if modified {
|
|
// At least one file in this build cycle has been modified
|
|
run(vars, "posthook")
|
|
modified = false
|
|
}
|
|
if !watch {
|
|
break
|
|
}
|
|
lastModified = time.Now()
|
|
time.Sleep(1 * time.Second)
|
|
}
|
|
}
|